libconfig_mex.h
00001 /* ---------------------------------------------------------------------------- 00002 00003 THE FILE IS UNDER THE CONSTRUCTION! 00004 00005 This file is a mex clone of libconfig.h++ originally found 00006 in the libconfig library which was desgigned by Mark A Lindner 00007 00008 ---------------------------------------------------------------------------- 00009 */ 00010 00011 #ifndef LIBCONFIG_MEX_H 00012 #define LIBCONFIG_MEX_H 00013 00014 #include <stdio.h> 00015 #include <string> 00016 #include <map> 00017 00018 00019 struct config_t; // fwd decl 00020 struct config_setting_t; // fwd decl 00021 00022 namespace libconfig { 00023 00024 class ConfigException : public std::exception { }; 00025 00026 class Setting; // fwd decl 00027 00028 class SettingException : public ConfigException 00029 { 00030 friend class Config; 00031 00032 public: 00033 00034 SettingException(const SettingException &other); 00035 SettingException& operator=(const SettingException &other); 00036 00037 virtual ~SettingException() throw(); 00038 00039 const char *getPath() const; 00040 00041 virtual const char *what() const throw(); 00042 00043 protected: 00044 00045 SettingException(const Setting &setting); 00046 SettingException(const Setting &setting, int idx); 00047 SettingException(const Setting &setting, const char *name); 00048 SettingException(const char *path); 00049 00050 private: 00051 00052 char *_path; 00053 }; 00054 00055 class SettingTypeException : public SettingException 00056 { 00057 friend class Config; 00058 friend class Setting; 00059 00060 public: 00061 00062 const char *what() const throw(); 00063 00064 private: 00065 00066 SettingTypeException(const Setting &setting); 00067 SettingTypeException(const Setting &setting, int idx); 00068 SettingTypeException(const Setting &setting, const char *name); 00069 }; 00070 00071 class SettingNotFoundException : public SettingException 00072 { 00073 friend class Config; 00074 friend class Setting; 00075 00076 public: 00077 00078 const char *what() const throw(); 00079 00080 private: 00081 00082 SettingNotFoundException(const Setting &setting, int idx); 00083 SettingNotFoundException(const Setting &setting, const char *name); 00084 SettingNotFoundException(const char *path); 00085 }; 00086 00087 class SettingNameException : public SettingException 00088 { 00089 friend class Config; 00090 friend class Setting; 00091 00092 public: 00093 00094 const char *what() const throw(); 00095 00096 private: 00097 00098 SettingNameException(const Setting &setting, const char *name); 00099 }; 00100 00101 class FileIOException : public ConfigException 00102 { 00103 public: 00104 00105 const char *what() const throw(); 00106 }; 00107 00108 class ParseException : public ConfigException 00109 { 00110 friend class Config; 00111 00112 public: 00113 00114 virtual ~ParseException() throw(); 00115 00116 inline const char *getFile() const throw() 00117 { return(_file); } 00118 00119 inline int getLine() const throw() 00120 { return(_line); } 00121 00122 inline const char *getError() const throw() 00123 { return(_error); } 00124 00125 const char *what() const throw(); 00126 00127 private: 00128 00129 ParseException(const char *file, int line, const char *error); 00130 00131 const char *_file; 00132 int _line; 00133 const char *_error; 00134 }; 00135 00136 class Setting 00137 { 00138 friend class Config; 00139 00140 public: 00141 00142 enum Type 00143 { 00144 TypeNone = 0, 00145 // scalar types 00146 TypeInt, 00147 TypeInt64, 00148 TypeFloat, 00149 TypeString, 00150 TypeBoolean, 00151 // aggregate types 00152 TypeGroup, 00153 TypeArray, 00154 TypeList 00155 }; 00156 00157 enum Format 00158 { 00159 FormatDefault = 0, 00160 FormatHex = 1 00161 }; 00162 00163 private: 00164 00165 config_setting_t *_setting; 00166 Type _type; 00167 Format _format; 00168 00169 Setting(config_setting_t *setting); 00170 00171 void assertType(Type type) const 00172 throw(SettingTypeException); 00173 static Setting & wrapSetting(config_setting_t *setting); 00174 00175 Setting(const Setting& other); // not supported 00176 Setting& operator=(const Setting& other); // not supported 00177 00178 public: 00179 00180 virtual ~Setting() throw(); 00181 00182 inline Type getType() const throw() { return(_type); } 00183 00184 inline Format getFormat() const throw() { return(_format); } 00185 void setFormat(Format format) throw(); 00186 00187 operator bool() const throw(SettingTypeException); 00188 operator int() const throw(SettingTypeException); 00189 operator unsigned int() const throw(SettingTypeException); 00190 operator long() const throw(SettingTypeException); 00191 operator unsigned long() const throw(SettingTypeException); 00192 operator long long() const throw(SettingTypeException); 00193 operator unsigned long long() const throw(SettingTypeException); 00194 operator double() const throw(SettingTypeException); 00195 operator float() const throw(SettingTypeException); 00196 operator const char *() const throw(SettingTypeException); 00197 operator std::string() const throw(SettingTypeException); 00198 00199 Setting & operator=(bool value) throw(SettingTypeException); 00200 Setting & operator=(int value) throw(SettingTypeException); 00201 Setting & operator=(long value) throw(SettingTypeException); 00202 Setting & operator=(const long long &value) throw(SettingTypeException); 00203 Setting & operator=(const double &value) throw(SettingTypeException); 00204 Setting & operator=(float value) throw(SettingTypeException); 00205 Setting & operator=(const char *value) throw(SettingTypeException); 00206 Setting & operator=(const std::string &value) throw(SettingTypeException); 00207 00208 Setting & operator[](const char * key) const 00209 throw(SettingTypeException, SettingNotFoundException); 00210 00211 inline Setting & operator[](const std::string & key) const 00212 throw(SettingTypeException, SettingNotFoundException) 00213 { return(operator[](key.c_str())); } 00214 00215 Setting & operator[](int index) const 00216 throw(SettingTypeException, SettingNotFoundException); 00217 00218 bool lookupValue(const char *name, bool &value) const throw(); 00219 bool lookupValue(const char *name, int &value) const throw(); 00220 bool lookupValue(const char *name, unsigned int &value) const throw(); 00221 bool lookupValue(const char *name, long long &value) const throw(); 00222 bool lookupValue(const char *name, unsigned long long &value) 00223 const throw(); 00224 bool lookupValue(const char *name, double &value) const throw(); 00225 bool lookupValue(const char *name, float &value) const throw(); 00226 bool lookupValue(const char *name, const char *&value) const throw(); 00227 bool lookupValue(const char *name, std::string &value) const throw(); 00228 00229 inline bool lookupValue(const std::string &name, bool &value) 00230 const throw() 00231 { return(lookupValue(name.c_str(), value)); } 00232 00233 inline bool lookupValue(const std::string &name, int &value) 00234 const throw() 00235 { return(lookupValue(name.c_str(), value)); } 00236 00237 inline bool lookupValue(const std::string &name, unsigned int &value) 00238 const throw() 00239 { return(lookupValue(name.c_str(), value)); } 00240 00241 inline bool lookupValue(const std::string &name, long long &value) 00242 const throw() 00243 { return(lookupValue(name.c_str(), value)); } 00244 00245 inline bool lookupValue(const std::string &name, 00246 unsigned long long &value) const throw() 00247 { return(lookupValue(name.c_str(), value)); } 00248 00249 inline bool lookupValue(const std::string &name, double &value) const 00250 throw() 00251 { return(lookupValue(name.c_str(), value)); } 00252 00253 inline bool lookupValue(const std::string &name, float &value) const 00254 throw() 00255 { return(lookupValue(name.c_str(), value)); } 00256 00257 inline bool lookupValue(const std::string &name, const char *&value) const 00258 throw() 00259 { return(lookupValue(name.c_str(), value)); } 00260 00261 inline bool lookupValue(const std::string &name, std::string &value) const 00262 throw() 00263 { return(lookupValue(name.c_str(), value)); } 00264 00265 void remove(const char *name) 00266 throw(SettingTypeException, SettingNotFoundException); 00267 00268 inline void remove(const std::string & name) 00269 throw(SettingTypeException, SettingNotFoundException) 00270 { remove(name.c_str()); } 00271 00272 void remove(unsigned int idx) 00273 throw(SettingTypeException, SettingNotFoundException); 00274 00275 inline Setting & add(const std::string & name, Type type) 00276 throw(SettingNameException, SettingTypeException) 00277 { return(add(name.c_str(), type)); } 00278 00279 Setting & add(const char *name, Type type) 00280 throw(SettingNameException, SettingTypeException); 00281 00282 Setting & add(Type type) throw(SettingTypeException); 00283 00284 inline bool exists(const std::string & name) const throw() 00285 { return(exists(name.c_str())); } 00286 00287 bool exists(const char *name) const throw(); 00288 00289 int getLength() const throw(); 00290 const char *getName() const throw(); 00291 std::string getPath() const; 00292 int getIndex() const throw(); 00293 00294 const Setting & getParent() const throw(SettingNotFoundException); 00295 Setting & getParent() throw(SettingNotFoundException); 00296 00297 bool isRoot() const throw(); 00298 00299 inline bool isGroup() const throw() 00300 { return(_type == TypeGroup); } 00301 00302 inline bool isArray() const throw() 00303 { return(_type == TypeArray); } 00304 00305 inline bool isList() const throw() 00306 { return(_type == TypeList); } 00307 00308 inline bool isAggregate() const throw() 00309 { return(_type >= TypeGroup); } 00310 00311 inline bool isScalar() const throw() 00312 { return((_type > TypeNone) && (_type < TypeGroup)); } 00313 00314 inline bool isNumber() const throw() 00315 { return((_type == TypeInt) || (_type == TypeInt64) 00316 || (_type == TypeFloat)); } 00317 00318 unsigned int getSourceLine() const throw(); 00319 const char *getSourceFile() const throw(); 00320 }; 00321 00322 class Config 00323 { 00324 private: 00325 00326 config_t *_config; 00327 00328 static void ConfigDestructor(void *arg); 00329 Config(const Config& other); // not supported 00330 Config& operator=(const Config& other); // not supported 00331 00332 public: 00333 00334 Config(); 00335 virtual ~Config(); 00336 00337 void setAutoConvert(bool flag); 00338 bool getAutoConvert() const; 00339 00340 void setTabWidth(unsigned short width) throw(); 00341 unsigned short getTabWidth() const throw(); 00342 00343 void read(FILE *stream) throw(ParseException); 00344 void write(FILE *stream) const; 00345 00346 void readString(const char *str) throw(ParseException); 00347 inline void readString(const std::string &str) throw(ParseException) 00348 { return(readString(str.c_str())); } 00349 00350 void readFile(const char *filename) throw(FileIOException, ParseException); 00351 void writeFile(const char *filename) throw(FileIOException); 00352 00353 inline Setting & lookup(const std::string &path) const 00354 throw(SettingNotFoundException) 00355 { return(lookup(path.c_str())); } 00356 00357 Setting & lookup(const char *path) const throw(SettingNotFoundException); 00358 00359 inline bool exists(const std::string & path) const throw() 00360 { return(exists(path.c_str())); } 00361 00362 bool exists(const char *path) const throw(); 00363 00364 bool lookupValue(const char *path, bool &value) const throw(); 00365 bool lookupValue(const char *path, int &value) const throw(); 00366 bool lookupValue(const char *path, unsigned int &value) const throw(); 00367 bool lookupValue(const char *path, long long &value) const throw(); 00368 bool lookupValue(const char *path, unsigned long long &value) 00369 const throw(); 00370 bool lookupValue(const char *path, double &value) const throw(); 00371 bool lookupValue(const char *path, float &value) const throw(); 00372 bool lookupValue(const char *path, const char *&value) const throw(); 00373 bool lookupValue(const char *path, std::string &value) const throw(); 00374 00375 inline bool lookupValue(const std::string &path, bool &value) const throw() 00376 { return(lookupValue(path.c_str(), value)); } 00377 00378 inline bool lookupValue(const std::string &path, int &value) const throw() 00379 { return(lookupValue(path.c_str(), value)); } 00380 00381 inline bool lookupValue(const std::string &path, unsigned int &value) 00382 const throw() 00383 { return(lookupValue(path.c_str(), value)); } 00384 00385 inline bool lookupValue(const std::string &path, long long &value) 00386 const throw() 00387 { return(lookupValue(path.c_str(), value)); } 00388 00389 inline bool lookupValue(const std::string &path, 00390 unsigned long long &value) const throw() 00391 { return(lookupValue(path.c_str(), value)); } 00392 00393 inline bool lookupValue(const std::string &path, double &value) 00394 const throw() 00395 { return(lookupValue(path.c_str(), value)); } 00396 00397 inline bool lookupValue(const std::string &path, float &value) 00398 const throw() 00399 { return(lookupValue(path.c_str(), value)); } 00400 00401 inline bool lookupValue(const std::string &path, const char *&value) 00402 const throw() 00403 { return(lookupValue(path.c_str(), value)); } 00404 00405 inline bool lookupValue(const std::string &path, std::string &value) 00406 const throw() 00407 { return(lookupValue(path.c_str(), value)); } 00408 00409 Setting & getRoot() const; 00410 00411 private: 00412 00413 void handleError() const; 00414 }; 00415 00416 }; // namespace libconfig 00417 00418 00419 #endif // LIBCONFIG_MEX_H
Generated on 2 Dec 2013 for mixpp by
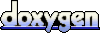