chmat.h
Go to the documentation of this file.00001 00013 #ifndef CHMAT_H 00014 #define CHMAT_H 00015 00016 #include "../bdmerror.h" 00017 #include "square_mat.h" 00018 00019 namespace bdm { 00020 00026 class chmat : public sqmat { 00027 protected: 00029 mat Ch; 00030 public: 00031 00032 void opupdt ( const vec &v, double w ); 00033 mat to_mat() const; 00034 void mult_sym ( const mat &C ); 00036 void mult_sym ( const mat &C , chmat &U ) const; 00037 void mult_sym_t ( const mat &C ); 00039 void mult_sym_t ( const mat &C, chmat &U ) const; 00040 double logdet() const; 00041 vec sqrt_mult ( const vec &v ) const; 00042 double qform ( const vec &v ) const; 00043 double invqform ( const vec &v ) const; 00044 void clear(); 00046 void add ( const chmat &A2, double w = 1.0 ); 00048 void inv ( chmat &Inv ) const { 00049 ( Inv.Ch ) = itpp::inv ( Ch ).T(); 00050 Inv.dim = dim; 00051 }; //Fixme: can be more efficient 00052 ; 00053 // void inv ( mat &Inv ); 00054 00056 virtual ~chmat() {}; 00058 chmat ( ) : sqmat (), Ch ( ) {}; 00060 chmat ( const int dim0 ) : sqmat ( dim0 ), Ch ( dim0, dim0 ) {}; 00062 chmat ( const vec &v ) : sqmat ( v.length() ), Ch ( diag ( sqrt ( v ) ) ) {}; 00064 chmat ( const chmat &Ch0 ) : sqmat ( Ch0.dim ), Ch ( Ch0.dim, Ch0.dim ) { 00065 Ch = Ch0.Ch; 00066 } 00067 00069 chmat ( const mat &M ) : sqmat ( M.rows() ), Ch ( M.rows(), M.cols() ) { 00070 mat Q; 00071 bdm_assert_debug ( M.rows() == M.cols(), "chmat:: input matrix must be square!" ); 00072 Ch = chol ( M ); 00073 } 00074 00080 chmat ( const chmat &M, const ivec &perm ): sqmat(perm.length()) { 00081 mat complete = M.to_mat (); 00082 mat subs = complete.get_cols(perm); 00083 Ch = chol(subs.get_rows(perm)); 00084 } 00085 00087 mat & _Ch() { 00088 return Ch; 00089 } 00091 const mat & _Ch() const { 00092 return Ch; 00093 } 00095 void setD ( const vec &nD ) { 00096 Ch = diag ( sqrt ( nD ) ); 00097 } 00099 void setCh ( const vec &chQ ) { 00100 bdm_assert_debug ( chQ.length() == dim * dim, "wrong length" ); 00101 copy_vector ( dim*dim, chQ._data(), Ch._data() ); 00102 } 00104 void setCh ( const chmat &Ch0 ) { 00105 Ch = Ch0._Ch(); 00106 dim = Ch0.rows(); 00107 } 00109 void setCh ( const mat &Ch0 ) { 00110 //TODO check if Ch0 is OK!!! 00111 Ch = Ch0; 00112 dim = Ch0.rows(); 00113 } 00114 00116 void setD ( const vec &nD, int i ) { 00117 for ( int j = i; j < nD.length(); j++ ) { 00118 Ch ( j, j ) = sqrt ( nD ( j - i ) ); //Fixme can be more general 00119 } 00120 } 00121 00123 chmat& operator += ( const chmat &A2 ); 00125 chmat& operator -= ( const chmat &A2 ); 00127 chmat& operator * ( const double &d ) { 00128 Ch*sqrt ( d ); 00129 return *this; 00130 }; 00132 chmat& operator = ( const chmat &A2 ) { 00133 Ch = A2.Ch; 00134 dim = A2.dim; 00135 return *this; 00136 } 00138 chmat& operator *= ( double x ) { 00139 Ch *= sqrt ( x ); 00140 return *this; 00141 }; 00142 }; 00143 00144 00147 inline chmat& chmat::operator += ( const chmat & A2 ) { 00148 this->add ( A2 ); 00149 return *this; 00150 } 00152 inline chmat& chmat::operator -= ( const chmat & A2 ) { 00153 this->add ( A2, -1.0 ); 00154 return *this; 00155 } 00156 00157 } 00158 00159 #endif // CHMAT_H
Generated on 2 Dec 2013 for mixpp by
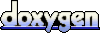