bdm::UI Class Reference
UI is an abstract class which collects all the auxiliary functions useful to prepare some concrete user-infos. More...
#include <user_info.h>
Inheritance diagram for bdm::UI:
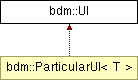
Public Types | |
optional | |
compulsory | |
enum | SettingPresence { optional, compulsory } |
Enum type used to determine whether the data for concrete Settingis is compulsory or optional. | |
Public Member Functions | |
virtual | ~UI () |
for future use | |
Static Public Member Functions | |
Initialization of classes | |
template<class T> | |
static bdm::shared_ptr< T > | build (const Setting &element, const string &name, SettingPresence settingPresence=optional) |
template<class T> | |
static bdm::shared_ptr< T > | build (const Setting &element, const int index, SettingPresence settingPresence=optional) |
template<class T> | |
static bdm::shared_ptr< T > | build (const Setting &element, SettingPresence settingPresence=optional) |
Initialization of structures | |
The type T has to be int, double, string, vec, ivec or mat. | |
template<class T> | |
static bool | get (T &instance, const Setting &element, const string &name, SettingPresence settingPresence=optional) |
template<class T> | |
static bool | get (T &instance, const Setting &element, const int index, SettingPresence settingPresence=optional) |
template<class T> | |
static bool | get (T &instance, const Setting &element) |
The existing instance of type T is initialized with values stored in the Setting element directly. | |
Initialization of arrays Array<T> | |
The type T has to be int, double, string, vec, ivec or mat, or pointer to any root descendant. | |
template<class T> | |
static bool | get (Array< T > &array_to_load, const Setting &element, const string &name, SettingPresence settingPresence=optional) |
template<class T> | |
static bool | get (Array< T > &array_to_load, const Setting &element, const int index, SettingPresence settingPresence=optional) |
template<class T> | |
static bool | get (Array< T > &array_to_load, const Setting &element) |
The existing array of type T is initialized with values stored in the Setting element. | |
Serialization of objects and structures into a new Setting | |
The new child Setting can be accessed either by its name - if some name is passed as a parameter - or by its integer index. In that case, the new element is added at the very end of the current list of child Settings. | |
static void | save (const root &instance, Setting &element, const string &name="") |
A root descendant is stored in the new child Setting appended to the passed element. | |
static void | save (const root *const instance, Setting &element, const string &name="") |
A pointer to root descendant is stored in the new child Setting appended to the passed element. | |
template<class T> | |
static void | save (const bdm::shared_ptr< T > &instance, Setting &element, const string &name="") |
A shared pointer to root descendant is stored in the new child Setting appended to the passed element. | |
template<class T> | |
static void | save (const Array< T > &array_to_save, Setting &element, const string &name="") |
An Array<T> instance is stored in the new child Setting appended to the passed element. | |
static void | save (const mat &matrix, Setting &element, const string &name="") |
A matrix(of type mat) is stored in the new child Setting appended to the passed element. | |
static void | save (const ldmat &matrix, Setting &element, const string &name="") |
A matrix(of type mat) is stored in the new child Setting appended to the passed element. | |
static void | save (const ivec &vec, Setting &element, const string &name="") |
An integer vector (of type ivec) is stored in the new child Setting appended to the passed element. | |
static void | save (const vec &vector, Setting &element, const string &name="") |
A double vector (of type vec) is stored in the new child Setting appended to the passed element. | |
static void | save (const string &str, Setting &element, const string &name="") |
A string is stored in the new child Setting appended to the passed element. | |
static void | save (const int &integer, Setting &element, const string &name="") |
An integer is stored in the new child Setting appended to the passed element. | |
static void | save (const double &real, Setting &element, const string &name="") |
A double is stored in the new child Setting appended to the passed element. | |
static void | save (const log_level_base &log_level, Setting &element) |
Protected Member Functions | |
UI (const string &class_name, const type_info *const class_type_info) | |
Constructor for internal use only, see. | |
Classes | |
class | MappedUI |
Detailed Description
UI is an abstract class which collects all the auxiliary functions useful to prepare some concrete user-infos.See static methods 'build', 'get' and 'save'. Writing user-infos with these methods is rather simple. The rest of this class is intended for internal purposes only. Its meaning is to allow pointers to its templated descendant ParticularUI<T>.
Constructor & Destructor Documentation
bdm::UI::UI | ( | const string & | class_name, | |
const type_info *const | class_type_info | |||
) | [inline, protected] |
Constructor for internal use only, see.
- See also:
- ParticularUI<T>
Member Function Documentation
static bdm::shared_ptr<T> bdm::UI::build | ( | const Setting & | element, | |
SettingPresence | settingPresence = optional | |||
) | [inline, static] |
The new instance of type T* is constructed and initialized with values stored in the given Setting
Handy in mex files. Use with care.
static bdm::shared_ptr<T> bdm::UI::build | ( | const Setting & | element, | |
const int | index, | |||
SettingPresence | settingPresence = optional | |||
) | [inline, static] |
The new instance of type T* is constructed and initialized with values stored in the Setting element[index]
If there does not exist any sub-element indexed by index, and settingPresence is optional, an empty bdm::shared_ptr<T> is returned. When settingPresence is compulsory, the returned bdm::shared_ptr<T> is never empty (an exception is thrown when the object isn't found).
static bdm::shared_ptr<T> bdm::UI::build | ( | const Setting & | element, | |
const string & | name, | |||
SettingPresence | settingPresence = optional | |||
) | [inline, static] |
The new instance of type T* is constructed and initialized with values stored in the Setting element[name]
If there does not exist any sub-element named name and settingPresence is optional, an empty bdm::shared_ptr<T> is returned. When settingPresence is compulsory, the returned bdm::shared_ptr<T> is never empty (an exception is thrown when the object isn't found).
static bool bdm::UI::get | ( | Array< T > & | array_to_load, | |
const Setting & | element, | |||
const int | index, | |||
SettingPresence | settingPresence = optional | |||
) | [inline, static] |
The existing array of type T is initialized with values stored in the Setting element[index] If there is not any sub-element indexed by index, this method returns false.
static bool bdm::UI::get | ( | Array< T > & | array_to_load, | |
const Setting & | element, | |||
const string & | name, | |||
SettingPresence | settingPresence = optional | |||
) | [inline, static] |
The existing array of type T is initialized with values stored in the Setting element[name] If there is not any sub-element named name, this method returns false.
static bool bdm::UI::get | ( | T & | instance, | |
const Setting & | element, | |||
const int | index, | |||
SettingPresence | settingPresence = optional | |||
) | [inline, static] |
The existing instance of type T is initialized with values stored in the Setting element[index] If there does not exist any sub-element indexed by index, this method returns false.
static bool bdm::UI::get | ( | T & | instance, | |
const Setting & | element, | |||
const string & | name, | |||
SettingPresence | settingPresence = optional | |||
) | [inline, static] |
The existing instance of type T is initialized with values stored in the Setting element[name] If there does not exist any sub-element named name, this method returns false.
The documentation for this class was generated from the following files:
- user_info.h
- user_info.cpp
Generated on 2 Dec 2013 for mixpp by
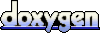