bdm::shared_ptr< T > Class Template Reference
A naive implementation of roughly a subset of the std::tr1::shared_ptr spec. More...
#include <shared_ptr.h>
Inheritance diagram for bdm::shared_ptr< T >:
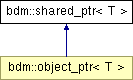
Public Member Functions | |
shared_ptr () | |
Default constructor. | |
shared_ptr (T *p) | |
shared_ptr (const shared_ptr< T > &other) | |
Copy constructor. | |
template<typename U> | |
shared_ptr (const shared_ptr< U > &other) | |
Generalized copy. | |
~shared_ptr () | |
shared_ptr< T > & | operator= (const shared_ptr< T > &other) |
Assignment operator. | |
T * | get () |
T * | operator-> () |
T & | operator * () |
const T * | get () const |
const T * | operator-> () const |
const T & | operator * () const |
bool | unique () const |
Returns use_count() == 1. | |
long | use_count () const |
operator bool () const | |
Boolean cast. | |
template<typename U> | |
operator shared_ptr () const | |
const cast | |
void | swap (shared_ptr &other) |
Efficient swap for shared_ptr. | |
Friends | |
class | shared_ptr |
Detailed Description
template<typename T>
class bdm::shared_ptr< T >
A naive implementation of roughly a subset of the std::tr1::shared_ptr spec.
Really just roughly - it ignores memory exceptions, for example; also note I didn't read the spec.
The standard template would naturally be preferable, if it was included in the standard libraries of all supported compilers - but as of 2009, that's still a problem...
Constructor & Destructor Documentation
bdm::shared_ptr< T >::shared_ptr | ( | ) | [inline] |
Default constructor.
Creates an empty shared_ptr - one that doesn't point anywhere.
bdm::shared_ptr< T >::shared_ptr | ( | T * | p | ) | [inline] |
Constructs a shared_ptr that owns the pointer p (unless p is NULL, in which case this constructor creates an empty shared_ptr). When p isn't null, it must have been alllocated by new!
bdm::shared_ptr< T >::shared_ptr | ( | const shared_ptr< T > & | other | ) | [inline] |
Copy constructor.
If other is empty, constructs an empty shared_ptr; otherwise, constructs a shared_ptr that shares ownership with other.
bdm::shared_ptr< T >::shared_ptr | ( | const shared_ptr< U > & | other | ) | [inline] |
Generalized copy.
Allows initialization of shared pointer of a base type from raw pointer to a derived type.
If other is empty, constructs an empty shared_ptr; otherwise, constructs a shared_ptr that shares ownership with other.
bdm::shared_ptr< T >::~shared_ptr | ( | ) | [inline] |
Destructor.
Member Function Documentation
const T* bdm::shared_ptr< T >::get | ( | ) | const [inline] |
Returns the stored pointer (which remains owned by this instance). For empty instances, this method returns NULL.
T* bdm::shared_ptr< T >::get | ( | ) | [inline] |
Returns the stored pointer (which remains owned by this instance). For empty instances, this method returns NULL.
const T& bdm::shared_ptr< T >::operator * | ( | ) | const [inline] |
Returns a reference to the object pointed to by the stored pointer. This method may only be called when the stored pointer isn't NULL.
T& bdm::shared_ptr< T >::operator * | ( | ) | [inline] |
Returns a reference to the object pointed to by the stored pointer. This method may only be called when the stored pointer isn't NULL.
bdm::shared_ptr< T >::operator bool | ( | ) | const [inline] |
Boolean cast.
This operator returns true if and only if the instance isn't empty.
bdm::shared_ptr< T >::operator shared_ptr | ( | ) | const [inline] |
const cast
Shared pointer to T can be converted to shared pointer to const T, just like T * can be converted to T const *.
const T* bdm::shared_ptr< T >::operator-> | ( | ) | const [inline] |
Returns the stored pointer (which remains owned by this instance). This method may only be called when the stored pointer isn't NULL.
T* bdm::shared_ptr< T >::operator-> | ( | ) | [inline] |
Dereferences the stored pointer (which remains owned by this instance). This method may only be called when the stored pointer isn't NULL.
long bdm::shared_ptr< T >::use_count | ( | ) | const [inline] |
Returns the number of shared_ptr instances (including this instance) that share ownership with this instance. For empty instances, this method returns 0.
The documentation for this class was generated from the following file:
Generated on 2 Dec 2013 for mixpp by
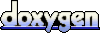