discrete.h
Go to the documentation of this file.00001 00013 #ifndef DISCR_H 00014 #define DISCR_H 00015 00016 00017 #include "../shared_ptr.h" 00018 #include "../base/bdmbase.h" 00019 #include "../math/chmat.h" 00020 00021 namespace bdm { 00022 00023 class support_base: public root { 00024 protected: 00026 int dim; 00028 int Npoints; 00030 vec actvec; 00032 double actvolume; 00033 public: 00035 support_base() : dim ( 0 ), Npoints ( 0 ) { 00036 } 00037 00039 virtual const vec& first_vec() NOT_IMPLEMENTED(empty_vec); 00040 00042 virtual const vec& next_vec() NOT_IMPLEMENTED(empty_vec);; 00043 00045 virtual const vec& act_vec() { 00046 return actvec; 00047 }; 00049 virtual const double& act_volume() { 00050 return actvolume; 00051 }; 00052 00054 int points() const { 00055 return Npoints; 00056 } 00057 00058 }; 00059 UIREGISTER ( support_base ); 00060 00064 class rectangular_support: public support_base { 00065 protected: 00067 Array<vec> ranges; 00069 ivec gridsizes; 00071 vec actvec_ind; 00072 // 00073 vec steps; 00074 public: 00076 rectangular_support() : support_base() { 00077 } 00078 00080 void set_parameters ( const Array<vec> &ranges0, const ivec &gridsize0 ); 00081 00083 void initialize(); 00084 00086 vec get_vec ( const ivec &inds ); 00087 00089 long linear_index ( const ivec inds ); 00090 00092 const vec& first_vec(); 00093 00095 const vec& next_vec(); 00096 00098 const vec& act_vec() { 00099 return actvec; 00100 }; 00101 00103 ivec nearest_point ( const vec &val ); 00104 00106 int points() const { 00107 return Npoints; 00108 } 00109 00112 const vec& _steps() const { 00113 return steps; 00114 } 00115 00116 void from_setting ( const Setting &set ); 00117 }; 00118 UIREGISTER ( rectangular_support ); 00119 00121 class discrete_support: public support_base { 00122 protected: 00124 Array<vec> Spoints; 00126 int idx; 00128 vec volumes; 00129 public: 00131 discrete_support() : Spoints ( 0 ), idx ( 0 ), volumes(0) {} 00133 int points() const { 00134 return Spoints.length(); 00135 } 00137 const vec& first_vec() { 00138 00139 bdm_assert_debug ( Spoints.length() > 0, "Empty support" ); 00140 idx = 0; 00141 actvolume=volumes(0); 00142 return Spoints ( idx ); 00143 } 00145 const vec& next_vec() { 00146 bdm_assert_debug ( Spoints.length() > idx - 1, "Out of support points" ); 00147 idx++; 00148 actvolume=volumes(idx); 00149 actvec = Spoints ( idx ); 00150 return actvec; 00151 } 00152 00162 void from_setting ( const Setting &set ); 00163 00164 void validate(){ 00165 bdm_assert(volumes.length()==Spoints.length(),"dimension mismatch"); 00166 Npoints=Spoints.length(); 00167 if (Npoints>0) 00168 dim = Spoints(0).length(); 00169 } 00171 Array<vec> & _Spoints() { 00172 return Spoints; 00173 } 00174 }; 00175 UIREGISTER ( discrete_support ); 00176 00178 class grid_fnc: public fnc { 00179 protected: 00181 shared_ptr<support_base> sup; 00183 vec values; 00184 public: 00186 void set_support ( shared_ptr<support_base> &sup0 ) { 00187 sup = sup0; 00188 values = zeros ( sup->points() ); 00189 } 00191 void set_values ( double ( *evalptr ) ( const vec& ) ); 00192 00194 void set_values ( const epdf &ep ); 00195 00197 double nearest_val ( const vec &val ) { 00198 NOT_IMPLEMENTED(0.0); 00199 // return values ( sup.linear_index ( sup.nearest_point ( val ) ) ); 00200 } 00201 00202 double integrate() ; 00203 00204 vec eval ( const vec &val ) { 00205 return vec_1 ( nearest_val ( val ) ); 00206 } 00207 const vec & _values() const { 00208 return values; 00209 } 00210 }; 00211 UIREGISTER ( grid_fnc ); 00212 00217 class egrid: public epdf { 00218 protected: 00220 rectangular_support sup; 00222 vec values; 00223 public: 00225 double evallog ( const vec &val ) { 00226 return log ( values ( sup.linear_index ( sup.nearest_point ( val ) ) ) ); 00227 } 00228 }; 00229 } 00230 #endif //DISCR_H
Generated on 2 Dec 2013 for mixpp by
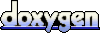