functions.h
00001 // 00002 // C++ Interface: itpp_ext 00003 // 00004 // Description: 00005 // 00006 // 00007 // Author: smidl <smidl@utia.cas.cz>, (C) 2008 00008 // 00009 // Copyright: See COPYING file that comes with this distribution 00010 // 00011 // 00012 #ifndef FN_H 00013 #define FN_H 00014 00015 #include "../bdmerror.h" 00016 #include "../base/bdmbase.h" 00017 00018 namespace bdm { 00019 00021 class constfn : public fnc { 00023 vec val; 00024 00025 public: 00026 //vec eval() {return val;}; 00028 vec eval ( const vec &cond ) { 00029 return val; 00030 }; 00032 constfn ( const vec &val0 ) : fnc(), val ( val0 ) { 00033 dimy = val.length(); 00034 dimc = 0; 00035 }; 00036 }; 00037 00039 class linfn: public fnc { 00040 public: 00042 RV rv; 00044 mat A; 00046 vec B; 00047 public : 00048 vec eval ( const vec &cond ) { 00049 bdm_assert_debug ( cond.length() == A.cols(), "linfn::eval Wrong cond." ); 00050 return A * cond + B; 00051 } 00052 00053 // linfn evalsome ( ivec &rvind ); 00055 linfn ( ) : fnc(), A ( ), B () { }; 00056 linfn ( const mat &A0, const vec &B0 ) : fnc(), A (A0 ), B (B0) { }; 00058 void set_parameters ( const mat &A0 , const vec &B0 ) { 00059 A = A0; 00060 B = B0; 00061 }; 00062 void from_setting(const Setting &set) { 00063 UI::get(A,set,"A",UI::compulsory); 00064 UI::get(B,set,"B",UI::compulsory); 00065 } 00066 void validate() { 00067 dimy = A.rows(); 00068 dimc = A.cols(); 00069 } 00070 00071 }; 00072 UIREGISTER(linfn); 00073 00074 00076 class quadraticfn: public fnc { 00077 public : 00079 RV rv; 00081 chmat Q; 00082 00084 quadraticfn ( ) : fnc(), Q ( ) { }; 00085 quadraticfn ( const mat &Q0) : fnc(), Q (Q0 ) { }; 00086 00087 vec eval ( const vec &cond ) { 00088 bdm_assert_debug ( cond.length() == Q.cols(), "quadraticfc::eval Wrong cond." ); 00089 return vec_1( Q.qform(concat(cond,1.0)) ); 00090 } 00091 00092 void from_setting(const Setting &set) { 00093 //UI::get(Q,set,"Q",UI::compulsory); 00094 } 00095 void validate() { 00096 dimy = 1; 00097 dimc = Q.cols()-1; 00098 } 00099 00100 }; 00101 UIREGISTER(quadraticfn); 00102 00112 class diffbifn: public fnc { 00113 protected: 00115 RV rvx; 00117 RV rvu; 00119 int dimx; 00121 int dimu; 00122 public: 00124 vec eval ( const vec &cond ) { 00125 bdm_assert_debug ( cond.length() == ( dimx + dimu ), "linfn::eval Wrong cond." ); 00126 if ( dimu > 0 ) { 00127 return eval ( cond ( 0, dimx - 1 ), cond ( dimx, dimx + dimu - 1 ) );//-1 = end (in matlab) 00128 } else { 00129 return eval ( cond ( 0, dimx - 1 ), vec ( 0 ) );//-1 = end (in matlab) 00130 } 00131 00132 } 00133 00135 virtual vec eval ( const vec &x0, const vec &u0 ) { 00136 return zeros ( dimy ); 00137 }; 00139 virtual void dfdx_cond ( const vec &x0, const vec &u0, mat &A , bool full = true ) {}; 00141 virtual void dfdu_cond ( const vec &x0, const vec &u0, mat &A, bool full = true ) {}; 00143 diffbifn () : fnc() {}; 00145 int _dimx() const { 00146 return dimx; 00147 } 00149 int _dimu() const { 00150 return dimu; 00151 } 00152 void validate(){dimc=dimx+dimu;}; 00153 }; 00154 00156 //TODO can be generalized into multilinear form! 00157 class bilinfn: public diffbifn { 00158 mat A; 00159 mat B; 00160 public : 00163 00164 bilinfn () : diffbifn (), A(), B() { } 00165 00166 bilinfn ( const mat &A0, const mat &B0 ) { 00167 set_parameters ( A0, B0 ); 00168 } 00169 00171 void set_parameters ( const mat &A0, const mat &B0 ) { 00172 bdm_assert ( A0.rows() == B0.rows(), "bilinfn matrices must have the same number of rows" ); 00173 A = A0; 00174 B = B0; 00175 dimy = A.rows(); 00176 dimx = A.cols(); 00177 dimu = B.cols(); 00178 } 00180 00183 inline vec eval ( const vec &x0, const vec &u0 ) { 00184 bdm_assert_debug ( x0.length() == dimx, "bilinfn::eval Wrong xcond." ); 00185 bdm_assert_debug ( u0.length() == dimu, "bilinfn::eval Wrong ucond." ); 00186 return dimu>0 ? A*x0 + B*u0 : A*x0; 00187 } 00188 00189 void dfdx_cond ( const vec &x0, const vec &u0, mat &F, bool full ) { 00190 bdm_assert_debug ( ( F.cols() == A.cols() ) && ( F.rows() == A.rows() ), "Allocated F is not compatible." ); 00191 if ( full ) F = A; //else : nothing has changed no need to regenerate 00192 } 00193 00194 void dfdu_cond ( const vec &x0, const vec &u0, mat &F, bool full = true ) { 00195 bdm_assert_debug ( ( F.cols() == B.cols() ) && ( F.rows() == B.rows() ), "Allocated F is not compatible." ); 00196 if ( full ) F = B; //else : nothing has changed no need to regenerate 00197 } 00198 void from_setting(const Setting &set) { 00199 diffbifn::from_setting(set); 00200 UI::get(A,set,"A",UI::compulsory); 00201 UI::get(B,set,"B",UI::compulsory); 00202 } 00203 void validate() { 00204 dimy = A.rows(); 00205 dimx = A.cols(); 00206 dimu = B.cols(); 00207 dimc =dimx+dimu; 00208 } 00210 }; 00211 UIREGISTER(bilinfn); 00212 00213 } //namespace 00214 #endif // FN_H
Generated on 2 Dec 2013 for mixpp by
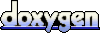