itpp_ext.h
00001 // 00002 // C++ Interface: itpp_ext 00003 // 00004 // Description: 00005 // 00006 // 00007 // Author: smidl <smidl@utia.cas.cz>, (C) 2008 00008 // 00009 // Copyright: See COPYING file that comes with this distribution 00010 // 00011 // 00012 #include <itpp/itbase.h> 00013 00014 #ifndef ITEX_H 00015 #define ITEX_H 00016 00017 using std::cout; 00018 using std::endl; 00019 00020 namespace itpp { 00021 extern vec empty_vec; 00022 00023 Array<int> to_Arr ( const ivec &indices ); 00024 ivec linspace ( int from, int to ); 00025 00026 vec get_vec ( const vec &v, const ivec &indexlist ); 00027 00028 bvec operator& ( const bvec &a, const bvec &b ); 00029 bvec operator| ( const bvec &a, const bvec &b ); 00030 00031 bvec operator> ( const vec &t1, const vec &t2 ); 00032 00033 bvec operator< ( const vec &t1, const vec &t2 ); 00034 00035 ivec get_from_bvec ( const ivec &v, const bvec &binlist ); 00036 00037 vec get_from_ivec ( const ivec &v); 00038 00039 // template<class Num_T> 00040 // void set_subvector(vec &ov, ivec &iv, const Vec<Num_T> &v); 00041 00042 void set_subvector ( vec &ov, const ivec &iv, const vec &v ); 00043 00044 template<class Num_T> inline 00045 void set_col_part ( mat &M, int c, const Vec<Num_T> &v ) { 00046 copy_vector ( v.size(), v._data(), M._data() + c*M.rows() ); 00047 } 00048 00049 static const double inf = std::numeric_limits<double>::infinity(); 00050 00051 #if 0 00052 00056 class Gamma_RNG { 00057 public: 00059 Gamma_RNG ( double a = 1.0, double b = 1.0 ); 00061 void setup ( double a0, double b0 ) { 00062 alpha = a0; 00063 beta = b0; 00064 } 00066 double get_setup() const; 00068 double operator() () { 00069 return sample(); 00070 } 00072 vec operator() ( int n ); 00074 mat operator() ( int h, int w ); 00075 protected: 00076 private: 00078 double sample(); 00080 double alpha; 00082 double beta; 00084 Random_Generator RNG; 00085 Normal_RNG NRNG; 00087 inline double exp_rand() { 00088 return -std::log ( RNG.random_01() ); 00089 } 00090 inline double unif_rand() { 00091 return RNG.random_01(); 00092 } 00093 inline double norm_rand() { 00094 return NRNG.sample(); 00095 } 00096 00097 }; 00098 bool qr ( const mat &A, mat &R ); 00099 #endif 00101 std::string num2str ( double d ); 00102 00104 std::string num2str ( int i ); 00105 00107 double psi ( double ); 00108 00110 void triu ( mat &A ); 00111 00115 double randun(); 00117 vec randun ( int n ); 00119 mat randun ( int n, int m ); 00120 00122 ivec unique ( const ivec &in ); 00123 00125 ivec unique_complement ( const ivec &in, const ivec &base ); 00126 } 00127 00128 00129 #endif //ITEX_H
Generated on 2 Dec 2013 for mixpp by
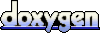