Using timers to measure execution time
In this example we are using the Real_Timer class to measure the execution time of a simple program. The Real_Timer class is included in the itmisc library.
#include <itpp/itbase.h> using namespace itpp; //These lines are needed for use of cout and endl using std::cout; using std::endl; int main() { //Declare the scalars used: long i, sum, N; //Declare tt as an instance of the timer class: Real_Timer tt; //Initiate the variables: N = 1000000; sum = 0; //Start and reset the timer: tt.tic(); //Do some processing for ( i = 0; i < N; i++ ) { sum += i; } // Print the elapsed time tt.toc_print(); //Print the result of the processing: cout << "The sum of all integers from 0 to " << N - 1 << " equals " << sum << endl; //Exit program: return 0; }
When you run this program, the output will look something like this:
Elapsed time = 0.000797055 seconds The sum of all integers from 0 to 999999 equals 1783293664
Generated on 2 Dec 2013 for mixpp by
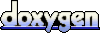