User Infos and their use
- See also:
- bdm::UI
UIREGISTER
Introduction
For easier interaction with users, experiments can be configured via structures called User Info. These structures contain information about details of the experiment that is to be performed. Since experiments involve the use of basic BDM classes and their compositions, the experiment description is also hierarchical with specific user info for each object or class.The User Infos are designed using customized version of the libconfig library (www.hyperrealm.com/libconfig). It is specialized for BDM so as to recognize basic mathematical objects, such as vectors and matrices, see details below.
Technically it can be made compatible with matlab structures.
- Todo:
- it will be good to create a more detailed paragraph about the intraction with Matlab
Example
For example, a simple experiment can be configures in the following way:ndat = 100; //number of data points prior = { class="enorm"; mu = [1, 2, 3]; R = [1, 0, 0, 0, 1, 0, 0, 0, 1]; };
ndat
and prior
) is defined by the application (or mex file) that is using this configuration file. It will look for expected fields and it will ignore any other structures. When it does not find what it is looking for, it terminates with an appropriate error message.
A structure with field class="identifier"
is special. Such a structure will be parsed by an appropriate bdm::UI::build method which will construct the desired object, in this instance of an object of the class bdm::enorm.
For a detailed example how this mechanism works in practice see Running experiment \c estimator with ARX data fields. To learn about the possinility of making internal or external links in configuration files, see the documentation of bdm::SettingResolver.
Implementation of user info in a custom class
In accordance with the previous example of the configuration file, consider the class defined as follows:
class newbee : public bdm::root { ... bdm::enorm prior; int ndat; ... }
There are few obligatory steps to implement user info mechanism in this class. At first, the class has to be inherited (directly or indirectly) from bdm::root, as you can see in our example. What is hidden behind the scene is the use of a parameterless constructor. Each class using User infos has to have one.
Next, two virtual methods bdm::root::from_setting and bdm::root::to_setting defined in the bdm::root class should be overriden in accordance with the content of the current class. In fact, you can override just method bdm::root::from_setting in the case you are interested only in loading from configuration files, which is quite common. It is valid also in the other way round, i.e., in the case you are interested just in saving, override only bdm::root::to_setting.
How should look the bodies of these methods? It is important not to forget to call their implementation in the base class (in our case, it is the bdm::root class). Then, they should contain loading of all the necessary class attributes in the case of bdm::root::from_setting method and saving of them in the other case. To implement these operation, you are encouraged to use static methods of bdm::UI class, namely bdm::UI::get, bdm::UI::build and bdm::UI::save.
It can look like this:
class newbee : public bdm::root { ... bdm::enorm prior; int ndat; ... virtual void from_setting(const Setting &set) { root::from_setting(set); if( !UI::get( ndat, set, "ndat" ) ) ndat = 1; prior = UI::build<enorm>( set, "prior", compulsory ); } virtual void to_setting(Setting &set) const { root::to_setting(set); UI::save(ndat, set, "ndat"); UI::save(prior, set, "prior"); } ... }
As you can see, the presence of a concrete Setting in the configuration file can be tested by the return value of these methods and the code initializing the default values can follow immediately. Imagine, for example, that the first attribute ndat
is optional. Thereore, the default value is filled in the case that there is not any other in the configuration file (and so bdm::UI::get method returs false
). The second atribute, prior
, is intended to be compulsory. This fact is specified by the last parameter of the templated bdm::UI::build method. In this case, the method throws an exception if there is not proper data in the configuration file.
The only difference between bdm::UI::build and bdm::UI::get method is in the types of variables they are prepared to. The bdm::UI::build<T> method is used to initialize instances of classes derived from bdm::root. It allocates them dynamically and return just an pointer to the new instance. This way it is possible even to load instances of inherited classes without aneven knowing about it. Oppositely, all scalar values of types int, double, string, vec, ivec or mat are loaded by the bdm::UI::get method with a static memory management. It is also capable to load arrays of templated type itpp::Array<T>
.
Saving is much more easier. For all the variable types, use the bdm::UI::save method.
Generated on 2 Dec 2013 for mixpp by
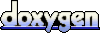