A very simple tutorial about vectors and matrixes
Let's start with a really simple example. Try to complile the following program:
#include <itpp/itbase.h> using namespace itpp; //These lines are needed for use of cout and endl using std::cout; using std::endl; int main() { //Declare vectors and matricies: vec a, b, c; mat A, B; //Use the function linspace to define a vector: a = linspace ( 1.0, 2.0, 10 ); //Use a string of values to define a vector: b = "0.1 0.2 0.3 0.4 0.5 0.6 0.7 0.8 0.9 1.0"; //Add two vectors: c = a + b; //Print results: cout << "a = " << a << endl; cout << "b = " << b << endl; cout << "c = " << c << endl; //Use a string to define a matrix: A = "1.0 2.0;3.0 4.0"; //Calculate the inverse of matrix A: B = inv ( A ); //Print results: cout << "A = " << A << endl; cout << "B = " << B << endl; //Exit program: return 0; }
When you run this program, the output shall look like this
a = [1 1.11111 1.22222 1.33333 1.44444 1.55556 1.66667 1.77778 1.88889 2] b = [0.1 0.2 0.3 0.4 0.5 0.6 0.7 0.8 0.9 1] c = [1.1 1.31111 1.52222 1.73333 1.94444 2.15556 2.36667 2.57778 2.78889 3] A = [[1 2] [3 4]] B = [[-2 1] [1.5 -0.5]]
If this is what you see, then congratulations! You have managed to compile your first it++ program!
Generated on 2 Dec 2013 for mixpp by
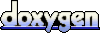